지우너
[코드트리] 주사위 던지기 C++ 본문
문제
https://www.codetree.ai/missions/2/problems/roll-a-dice?&utm_source=clipboard&utm_medium=text
코드트리 | 코딩테스트 준비를 위한 알고리즘 정석
국가대표가 만든 코딩 공부의 가이드북 코딩 왕초보부터 꿈의 직장 코테 합격까지, 국가대표가 엄선한 커리큘럼으로 준비해보세요.
www.codetree.ai
계획세우기
r, c에서 시작
dx, dy를 이용하여 좌표 이동
이동 방향에 따라 주사위 굴리기
→ 주사위의 3면을 저장하는 dice 배열을 만든다. arr에 저장되는 값은 아랫면이기 때문에 arr[x][y]=7-dice[0]을 해주면 된다.
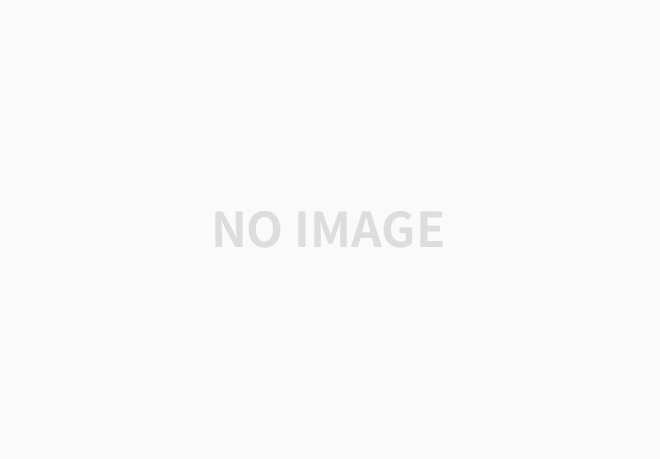
풀이
풀고 나서 보니 굳이 char로 들어오는 입력을 int로 바꿔서 저장할 필요는 없었을 것 같다. 길이도 길어지고 가독성도 구려진 느낌...?
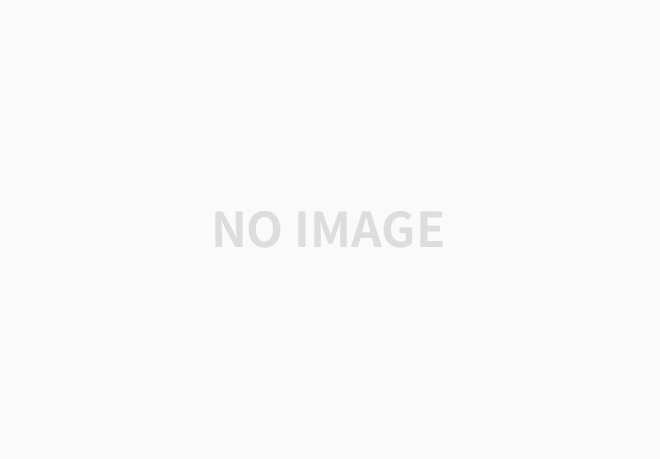
#include <iostream>
using namespace std;
int n, m, r, c;
int arr[101][101];
int dirNum[10001];
// arr 좌표 안에 들어갈 수 관리
int dice[3]={1, 2, 3}; // 위 정면 오른쪽
int CharDirToInt(char d){
if (d=='L') return 0;
else if (d=='U') return 1;
else if (d=='R') return 2;
else return 3;
}
void RollTheDice(int d){
if(d==0){
int tmp = dice[0];
dice[0]=dice[2];
dice[2]=7-tmp;
}
else if(d==1){
int tmp = dice[0];
dice[0]=dice[1];
dice[1]=7-tmp;
}
else if(d==2){
int tmp = dice[2];
dice[2]=dice[0];
dice[0]=7-tmp;
}
else {
int tmp = dice[1];
dice[1]=dice[0];
dice[0]=7-tmp;
}
}
void Play(){
// arr좌표를 움직이기 위한 dx dy
// 좌(0,-1) 상(-1,0) 우(0,1) 하(1,0)
int dx[4]={0, -1, 0, 1};
int dy[4]={-1, 0, 1, 0};
arr[r][c] = 7-dice[0];
for(int i=0; i<m; ++i){
int nx=r+dx[dirNum[i]];
int ny=c+dy[dirNum[i]];
if(nx<0 || nx>=n || ny<0 || ny>=n) continue;
RollTheDice(dirNum[i]);
r=nx;
c=ny;
arr[r][c] = 7-dice[0];
}
}
int main() {
cin >> n >> m >> r >> c;
r-=1;
c-=1;
for(int i=0; i<m; ++i){
char d;
cin >> d;
dirNum[i]=CharDirToInt(d);
}
Play();
int answer =0;
for(int i=0; i<n; ++i){
for(int j=0; j<n; ++j){
answer+=arr[i][j];
}
}
cout << answer << '\n';
return 0;
}
'Problem Solving' 카테고리의 다른 글
[코드트리] 핀볼게임 C++ (0) | 2024.06.17 |
---|---|
[프로그래머스 Lv.0] 평행 C++ (0) | 2024.06.14 |
[코드트리] 최적의 십자 모양 폭발 C++ (0) | 2024.06.13 |
[코드트리] 2차원 폭발 게임 C++ (0) | 2024.06.12 |
[코드트리] 십자 모양의 지속적 폭발 C++ (0) | 2024.06.11 |